PHP 8 will add union types, the JIT, attributes/annotations, throw expressions, weak maps, trailing parameter list commas, a Stringable interface, strstartswith, strendswith and strcontains functions, and so much more — thanks to everyone working on this release!
Specialisation of the Archive class to allow reading of files from a zip format source archive. More..
#include <OgreZip.h>
Public Member Functions |
ZipArchive (const String &name, const String &archType, zzip_plugin_io_handlers *pluginIo=NULL) |
~ZipArchive () |
DataStreamPtr | create (const String &filename) |
Create a new file (or overwrite one already there). More..
|
bool | exists (const String &filename) const |
Find out if the named file exists (note: fully qualified filename required) More..
|
StringVectorPtr | find (const String &pattern, bool recursive=true, bool dirs=false) const |
Find all file or directory names matching a given pattern in this archive. More..
|
FileInfoListPtr | findFileInfo (const String &pattern, bool recursive=true, bool dirs=false) const |
Find all files or directories matching a given pattern in this archive and get some detailed information about them. More..
|
time_t | getModifiedTime (const String &filename) const |
Retrieve the modification time of a given file. More..
|
const String & | getName (void) const |
Get the name of this archive. More..
|
const String & | getType (void) const |
Return the type code of this Archive. More..
|
bool | isCaseSensitive (void) const |
Returns whether this archive is case sensitive in the way it matches files. More..
|
virtual bool | isReadOnly () const |
Reports whether this Archive is read-only, or whether the contents can be updated. More..
|
StringVectorPtr | list (bool recursive=true, bool dirs=false) const |
List all file names in the archive. More..
|
FileInfoListPtr | listFileInfo (bool recursive=true, bool dirs=false) const |
List all files in the archive with accompanying information. More..
|
void | load () |
Loads the archive. More..
|
DataStreamPtr | open (const String &filename, bool readOnly=true) const |
Open a stream on a given file. More..
|
void | remove (const String &filename) |
Delete a named file. More..
|
void | unload () |
Unloads the archive. More..
|
Detailed Description
Specialisation of the Archive class to allow reading of files from a zip format source archive.
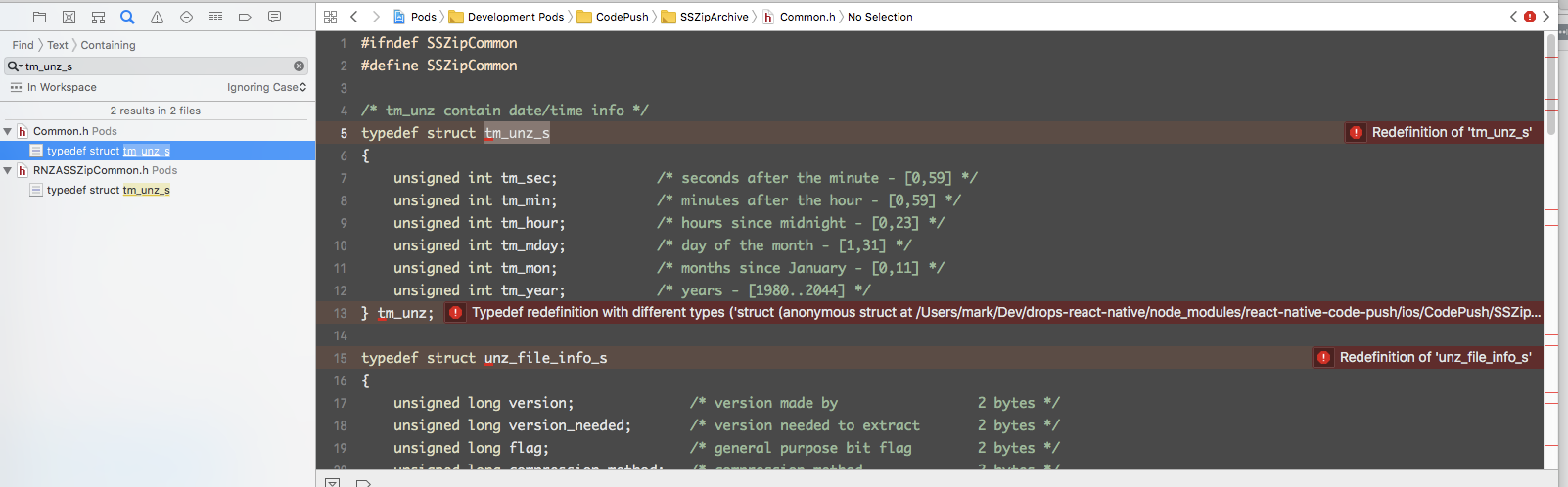
- Remarks
- This archive format supports all archives compressed in the standard zip format, including iD pk3 files.
Constructor & Destructor Documentation
◆ ZipArchive()
Ogre::ZipArchive::ZipArchive | ( | const String & | name, |
const String & | archType, |
zzip_plugin_io_handlers * | pluginIo = NULL |
) |
◆ ~ZipArchive()
Member Function Documentation
◆ isCaseSensitive()
bool Ogre::ZipArchive::isCaseSensitive | ( | void | ) | const |
| inlinevirtual |
Returns whether this archive is case sensitive in the way it matches files.
Implements Ogre::Archive.
◆ load()
Loads the archive.
- Remarks
- This initializes all the internal data of the class.
- Warning
- Do not call this function directly, it is meant to be used only by the ArchiveManager class.
Implements Ogre::Archive.
◆ unload()
Unloads the archive. Essential anatomy 5 for pc.
- Warning
- Do not call this function directly, it is meant to be used only by the ArchiveManager class.
Implements Ogre::Archive.
◆ open()
DataStreamPtr Ogre::ZipArchive::open | ( | const String & | filename, | bool | readOnly = true | ) | const |
| virtual |
Open a stream on a given file.
- Note
- There is no equivalent 'close' method; the returned stream controls the lifecycle of this file operation.
Parametersfilename | The fully qualified name of the file |
readOnly | Whether to open the file in read-only mode or not (note, if the archive is read-only then this cannot be set to false) |
- Returns
- A shared pointer to a DataStream which can be used to read / write the file. If the file is not present, returns a null shared pointer.
Implements Ogre::Archive.
◆ create()
DataStreamPtr Ogre::ZipArchive::create | ( | const String & | filename | ) |
| virtual |
Create a new file (or overwrite one already there).

- Note
- If the archive is read-only then this method will fail.
Parametersfilename | The fully qualified name of the file |
- Returns
- A shared pointer to a DataStream which can be used to read / write the file.
Reimplemented from Ogre::Archive.
◆ remove()
void Ogre::ZipArchive::remove | ( | const String & | filename | ) |
| virtual |
Delete a named file. Getnet gn 531u drivers for mac free.
- Remarks
- Not possible on read-only archives
Parametersfilename | The fully qualified name of the file |
Reimplemented from Ogre::Archive.
◆ list()
StringVectorPtr Ogre::ZipArchive::list | ( | bool | recursive = true , | bool | dirs = false | ) | const |
| virtual |
List all file names in the archive.
- Note
- This method only returns filenames, you can also retrieve other information using listFileInfo.
Parametersrecursive | Whether all paths of the archive are searched (if the archive has a concept of that) |
dirs | Set to true if you want the directories to be listed instead of files |
- Returns
- A list of filenames matching the criteria, all are fully qualified
Implements Ogre::Archive.
◆ listFileInfo()
FileInfoListPtr Ogre::ZipArchive::listFileInfo | ( | bool | recursive = true , | bool | dirs = false | ) | const |
| virtual |
List all files in the archive with accompanying information.
Parametersrecursive | Whether all paths of the archive are searched (if the archive has a concept of that) |
dirs | Set to true if you want the directories to be listed instead of files |
- Returns
- A list of structures detailing quite a lot of information about all the files in the archive.
Implements Ogre::Archive.
◆ find()
StringVectorPtr Ogre::ZipArchive::find | ( | const String & | pattern, | bool | recursive = true , | bool | dirs = false | ) | const |
| virtual |
Find all file or directory names matching a given pattern in this archive.
- Note
- This method only returns filenames, you can also retrieve other information using findFileInfo.
Parameterspattern | The pattern to search for; wildcards (*) are allowed |
recursive | Whether all paths of the archive are searched (if the archive has a concept of that) |
dirs | Set to true if you want the directories to be listed instead of files |
- Returns
- A list of filenames matching the criteria, all are fully qualified
Implements Ogre::Archive.
◆ findFileInfo()
FileInfoListPtr Ogre::ZipArchive::findFileInfo | ( | const String & | pattern, | bool | recursive = true , | bool | dirs = false | ) | const |
| virtual |
Find all files or directories matching a given pattern in this archive and get some detailed information about them.
Parameterspattern | The pattern to search for; wildcards (*) are allowed |
recursive | Whether all paths of the archive are searched (if the archive has a concept of that) |
dirs | Set to true if you want the directories to be listed instead of files |
- Returns
- A list of file information structures for all files matching the criteria.
Implements Ogre::Archive.
◆ exists()
bool Ogre::ZipArchive::exists | ( | const String & | filename | ) | const |
| virtual |
Find out if the named file exists (note: fully qualified filename required)
Implements Ogre::Archive.
◆ getModifiedTime()
time_t Ogre::ZipArchive::getModifiedTime | ( | const String & | filename | ) | const |
| virtual |
Retrieve the modification time of a given file.
Implements Ogre::Archive.
◆ getName()
const String& Ogre::Archive::getName | ( | void | ) | const |
| inlineinherited |
◆ isReadOnly()
virtual bool Ogre::Archive::isReadOnly | ( | ) | const |
| inlinevirtualinherited |
Reports whether this Archive is read-only, or whether the contents can be updated.
References Ogre::FileInfo::filename.
◆ getType()
const String& Ogre::Archive::getType | ( | void | ) | const |
| inlineinherited |
Return the type code of this Archive.
Referenced by Ogre::APKFileSystemArchiveFactory::createInstance(), and Ogre::APKFileSystemArchiveFactory::~APKFileSystemArchiveFactory().
The documentation for this class was generated from the following file: